Object-oriented programming Learn web development MDN
Table Of Content
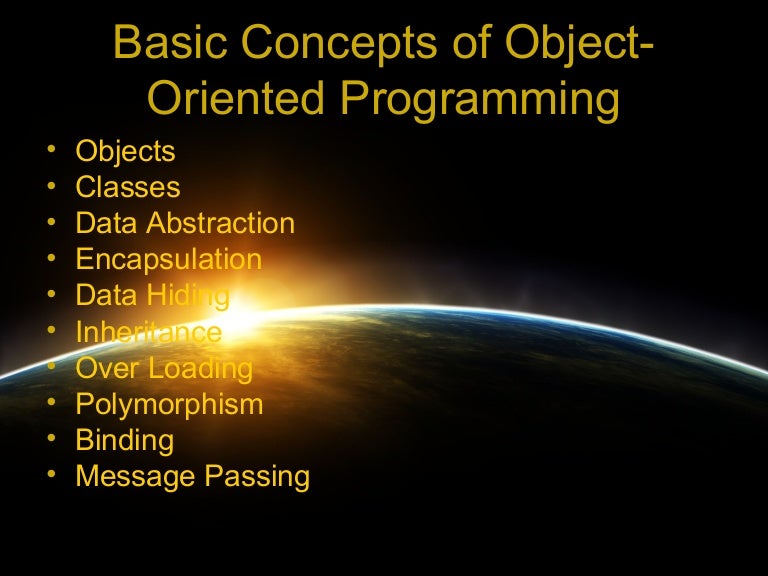
Ahead, we’ll break down what you need to know about object-oriented programming and the courses to take if you want to get started. In this tutorial, you learned about object-oriented programming (OOP) in Python. Most modern programming languages, such as Java, C#, and C++, follow OOP principles, so the knowledge that you gained here will be applicable no matter where your programming career takes you. It’s a higher-level pattern compared to the Factory Method Pattern, where you have not just one factory but a family of factories that work together to create related objects.
What is Spring Framework? Definition from TechTarget - TechTarget
What is Spring Framework? Definition from TechTarget.
Posted: Mon, 24 Jan 2022 23:05:01 GMT [source]
Objects and Classes
Object-oriented is the most popular, and the one most often taught in programming courses. Every programming language has its own syntax and features, and many of them also use different paradigms or styles of writing and organizing code. In essence, OOP is a powerful paradigm for creating organized, flexible, and efficient code, making software development more manageable and rewarding. Encapsulation and Data Hiding are two fundamental principles of object-oriented programming.
Object Model Object Oriented Analysis & Design
When a subclass is instantiated, a single object is created which combines properties defined in the subclass with properties defined further up the hierarchy. With prototyping, each level of the hierarchy is represented by a separate object, and they are linked together via the __proto__ property. The prototype chain's behavior is less like inheritance and more like delegation. Delegation is a programming pattern where an object, when asked to perform a task, can perform the task itself or ask another object (its delegate) to perform the task on its behalf. In many ways, delegation is a more flexible way of combining objects than inheritance (for one thing, it's possible to change or completely replace the delegate at run time). Mastering OOP involves not just understanding the theory behind it but also adopting the best practices and design patterns that make your code efficient, reusable, and easy to maintain.
The SOLID Principles of Object-Oriented Programming Explained in Plain English
“Public” dictates that the attributes and methods can be accessed, modified, or executed from the outside. This means that classes that inherit from the parent class can also access these attributes and methods, just like the parent class. Inheritance allows a class to inherit the features of other classes. The original class is called the parent class, and the class inheriting the features is called the child class.
Encapsulation is the concept of bundling data (attributes or variables) and the methods (functions) that operate on that data into a single unit called a class. It restrict access to some of an object’s components, providing a way to control and protect data from external inteference. Encapsulation helps achieve data abstraction and maintain the integrity of an object’s state.
What is Unified Modeling Language (UML)? Definition from TechTarget - TechTarget
What is Unified Modeling Language (UML)? Definition from TechTarget.
Posted: Tue, 15 Mar 2022 02:37:10 GMT [source]
A class serves as a high-level blueprint for generating more specific, concrete objects. Classes typically represent general categories, like Bicycle or Book, which have common attributes. These classes define the attributes that an object of this type will possess, such as color, brand, but not the specific values for those attributes in a given object.

Four Pillars of OOP
Objects provide an interface to other code that wants to use them but maintain their own internal state. The object's internal state is kept private, meaning that it can only be accessed by the object's own methods, not from other objects. Keeping an object's internal state private, and generally making a clear division between its public interface and its private internal state, is called encapsulation. In recent years, object-oriented programming has become especially popular in dynamic programming languages.
To do this, we can create a fly() method in the child class that overrides the fly() method in the parent IronMan class. Encapsulation enhances code security and makes collaboration with external developers easier. When sharing information with another company, it's important not to expose class templates or private data since they are part of your company's intellectual property. In our IronMan’s class example, the constructor sets up the initial state of the Iron Man object with name, suit color, and armor strength. Note that JavaScript does not have destructors like some other languages. Instead, memory management and resource cleanup are handled by the garbage collector.
Design Patterns
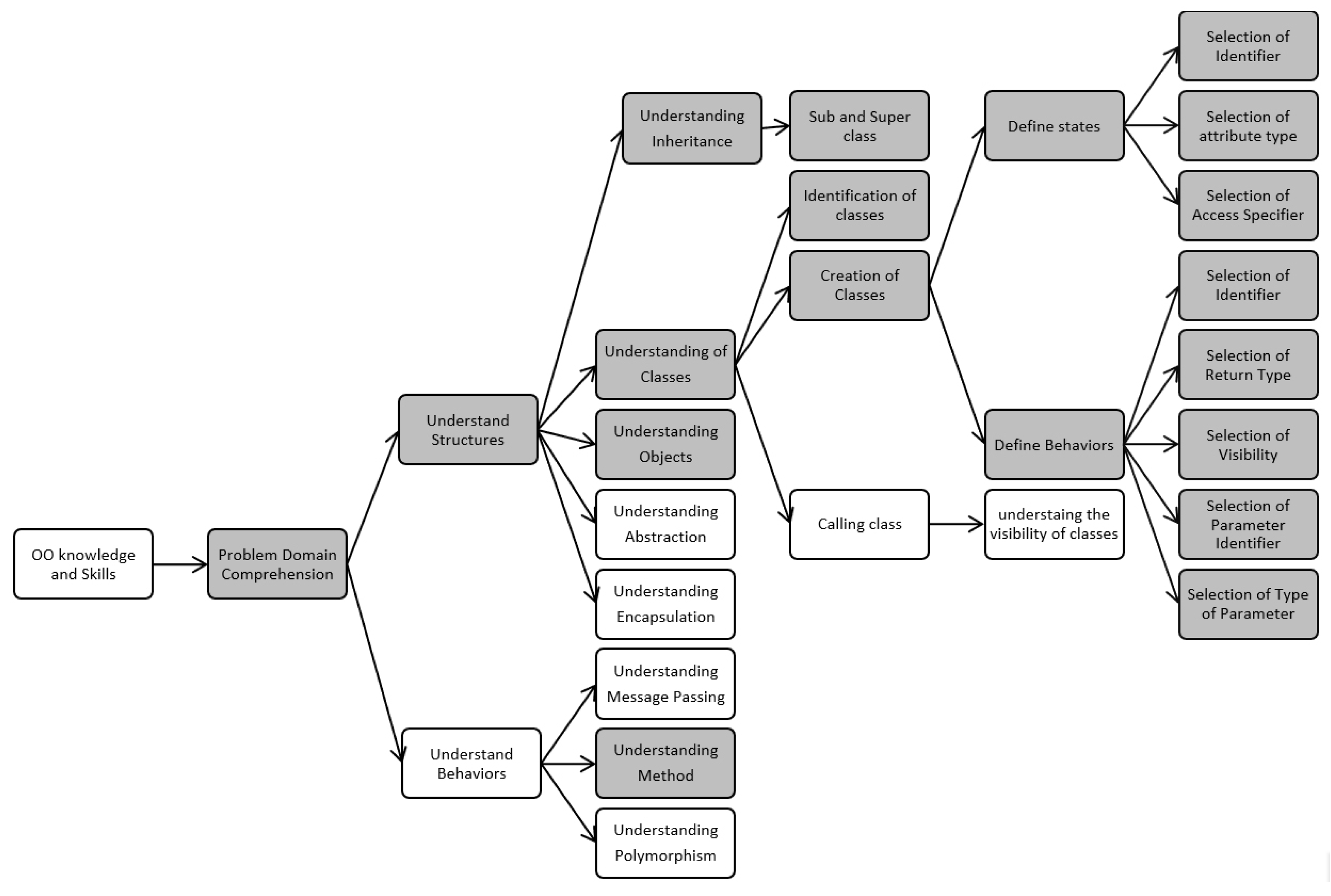
When two types share an inheritance chain, they can be used interchangeably with no errors or assertions in your code. Developers have a principle called the Liskov Substitution principle. It states that if you can use a parent class (let's call it ParentType) anywhere you use a child (let's call it ChildType) – and ChildType inherits from the ParentType – then you pass the test. Encapsulation means that each object in your code should control its own state. The keys, the methods on your object, Boolean properties and so on. If you were to reset a Boolean or delete a key from the object, they're all changes to your state.
You wanted a banana but what you got was a gorilla holding the banana and the entire jungle. With this new model, we can even go further and split the PaidParkingLot to support different types of payment. Now consider that we want to implement a parking lot that is free. In the first test, we create a rectangle where the width is 2 and the height is 3 and call getAreaTest. The output is 20 as expected, but things go wrong when we pass in the square. This is because the call to setHeight function in the test is setting the width as well and results in an unexpected output.
Child classes can override or extend the attributes and methods of parent classes. In other words, child classes inherit all of the parent’s attributes and methods but can also specify attributes and methods that are unique to themselves. This principle helps us to implement flexible and maintainable code. It states that we should have to implement interfaces rather than extending the classes. We implement the inheritance when the class need to implement all the functionalities and the child class can be used as a substitute for our parent class.
This isn't abstracted away as you need to worry about the implementation for every new type you add. JavaScript is a multi-paradigm language and can be written following different programming paradigms. A programming paradigm is essentially a bunch of rules that you follow when writing code, to help you solve a particular problem. In this example the Visitor Pattern allows you to add new tax calculation functionality to your finance application without modifying the existing classes of taxable items. Each taxable item can accept the tax calculator (visitor) and delegate the tax calculation operation on it. The Template Method Pattern is used in scenarios where you want to define the outline of an algorithm and allows subclasses to provide specific implementations for certain steps.
In the mid-1980s Objective-C was developed by Brad Cox, who had used Smalltalk at ITT Inc.. Bjarne Stroustrup, who had used Simula for his PhD thesis, created the object-oriented C++.[13] In 1985, Bertrand Meyer also produced the first design of the Eiffel language. Focused on software quality, Eiffel is a purely object-oriented programming language and a notation supporting the entire software lifecycle.
In this tutorial, you’ll learn the basics of object-oriented programming in Python. The Template Method Pattern is a behavioral desing pattern in object-oriented programming. It defines the skeleton of an algorithm in a method, deferring some steps to subclasses. It allows subclasses to redefine certain steps of an algorithm wihout altering the algorithm’s structure.
When you call the HTML method on the $output2 object, you will get an E_NOTICE error, informing you of an array-to-string conversion. Then q(y) should be provable for objects y of type S where S is a subtype of T. Now, you can create another shape class and pass it in when calculating the sum without breaking the code. A way you can make this sum method better is to remove the logic to calculate the area of each shape out of the AreaCalculator class method and attach it to each shape’s class. A class should have one and only one reason to change, meaning that a class should have only one job. In this article, you will be introduced to each principle individually to understand how SOLID can help make you a better developer.
Object-Oriented Programming (OOP) is a game-changing and popular programming paradigm that streamlines software design and development by centering on objects, their unique qualities and actions. Almost every developer deal with OOP at some point in their career so it’s quite essential to master OOP concepts. Additional design principles will help you to create code that is flexible, reusable, and maintainable. In this module you will learn about coupling and cohesion, separation of concerns, information hiding, and conceptual integrity. You will also learn to avoid common pitfalls with inheritance, and ways to express software behavior in UML. First, in class-based OOP, classes and objects are two separate constructs, and objects are always created as instances of classes.
Comments
Post a Comment