Design Patterns
Table Of Content
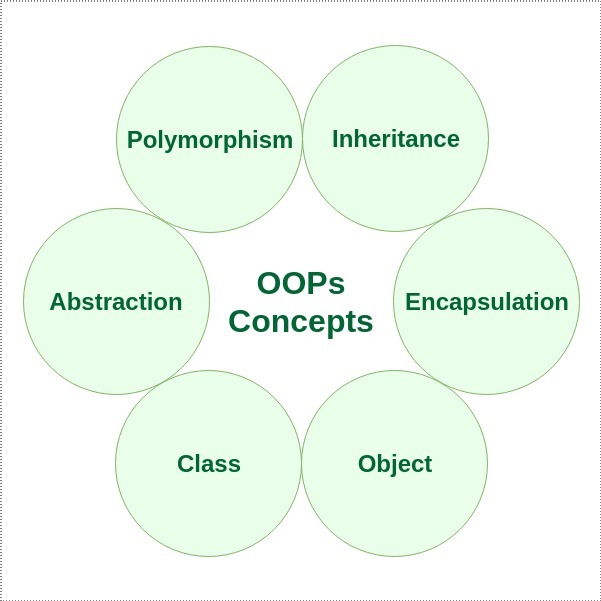
The principle was introduced by Barbara Liskov in 1987 and according to this principle “Derived or child classes must be substitutable for their base or parent classes“. It applies to inheritance hierarchies, specifying that you should design your classes so that client dependencies can be substituted with subclasses without the client knowing about the change. The Decorator Pattern is a structural desing pattern in object-oriented programming. It allows you to dynamically add new behavior or responsibilities to objects without altering their code. This pattern is acheived by creating a set of decorator classes that are used to wrap the original object. Each decorator addis its own functionality while delegating the rest of the work to the wrapped object.
Explain OOP Like I'm 5
Having to manage all of those other things while driving the car would cause chaos and a lot of accidents. This represents an aggregation, where a school is composed of students. Aggregation is a type of association that represnets a whole-part relationship. It implies that one one class (the whole) contians or is composed of another class (the part). Give the sound argument of GoldenRetriever.speak() a default value of "Bark".
Data Hiding
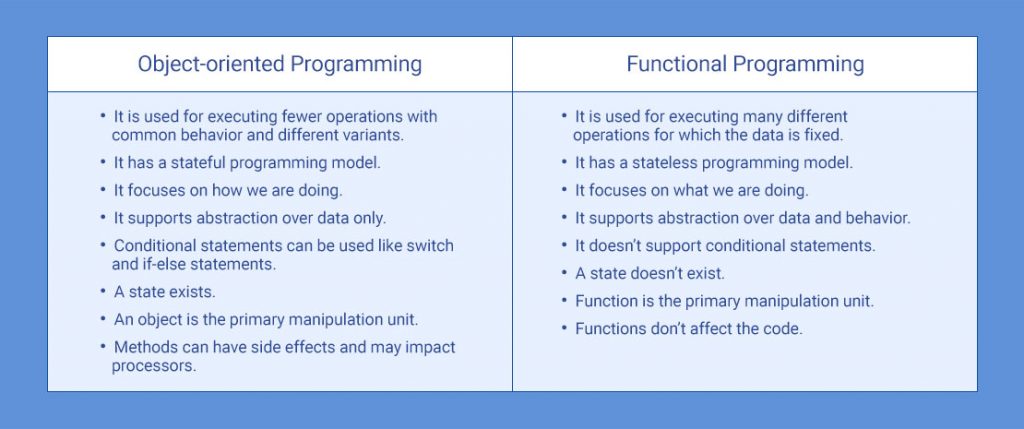
Dispatch interacts with inheritance; if a method is not present in a given object or class, the dispatch is delegated to its parent object or class, and so on, going up the chain of inheritance. Data Abstraction – Abstraction refers to the act of representing important and special features without including the background details or explanation about that feature. We want our classes to be open to extension, so we have reorganized our dependencies to depend on interfaces instead of concrete classes.
Learn Python 3
Designers May Find C++ to Their Liking - EE Times
Designers May Find C++ to Their Liking.
Posted: Fri, 06 Dec 2019 22:02:24 GMT [source]
Modules are namespaced so identifiers in one module will not conflict with a procedure or variable sharing the same name in another file or module. Polymorphism – Polymorphism is the ability of data to be processed in more than one form. It consists of method overloading and method overriding, i.e., writing the method once and performing a number of tasks using the same method name.
Data Engineers Shouldn't Write Airflow Dags — Part 2 - Towards Data Science
Data Engineers Shouldn't Write Airflow Dags — Part 2.
Posted: Wed, 16 Jun 2021 07:00:00 GMT [source]
New to Software Development? Start here.
So when we create a class, we do not need to write all the properties and functions again and again, as these can be inherited from another class that possesses it. Inheritance allows the user to reuse the code whenever possible and reduce its redundancy. Second, although a prototype chain looks like an inheritance hierarchy and behaves like it in some ways, it's different in others.

New objects can be created based on already existing objects chosen as their prototype. You may call two different objects apple and orange a fruit if the object fruit exists, and both apple and orange have fruit as their prototype. Terminology invoking "objects" in the modern sense of object-oriented programming made its first appearance at the artificial intelligence group at MIT in the late 1950s and early 1960s. Classes are user-defined data types used as a blueprint or template for the objects a software program will use in its operation. Classes define the methods and attributes that each object created from them has. Most object-oriented programming languages use classes, though some (like Go, for example) do not.
Encapsulation and Data Hiding
The Observer Pattern is used in various scenarios where a one-to-many relationship exists between objects, and one subject needs to notify multiple others about changes. This is analogous to how a proxy in software provide caching, lazy loading, or access control without affecting the underlying object. The Abstract Factory Pattern is used when you need to ensure that the created objects are compatible and belong to a consistent family. The Factory Method Pattern is used in software development when you need to decouple the object creation from the object’s usage. By encapsulating data, you make the information of your system safer and more reliable. You're also able monitor how the information is being accessed and what operations are performed on it.
Object-oriented concepts
The remote control interacts with commands that encapsualte specific actions, providing a more flexible and decoupled way to control the TV. The Strategy Pattern is used in situations where multiple algorithms or strategies are available for a specific task, and you want to select and use one of them at runtime. For example, you'll define an employee object that defines all the overall characteristics of employees in your company. You do this by hiding details that aren't necessary for the user to see.
How Do You Instantiate a Class in Python?
Just like in a house, it's important to have public interfaces to share information that others need, like the address or the house's exterior appearance. But it's also important to keep private information hidden, like the family's daily routines or the location of valuable belongings. If we shared that private information, it might make guests uncomfortable or put the family's safety at risk.
This pattern promotes reusability by providing a common structure for a family of related algorithms. If you were to conduct a fast internet search on what object-oriented programming is, you'll find that OOP is defined as a programming paradigm that relies on the concept of classes and objects. On the other side, OOP, which offers a different perspective on organizing code.
In languages that support open recursion, object methods can call other methods on the same object (including themselves) using this name. This variable is late-bound; it allows a method defined in one class to invoke another method that is defined later, in some subclass thereof. Here, we’ve created two Car objects myCar and yourCar, and accessed their attributes and methods. Each object has its own set of attributes and can have its methods called independently. You define the properties that all Dog objects must have in a method called .__init__().
To do this, you still need to define a .speak() method on the child JackRussellTerrier class. You’ll learn more about how the code above works in the sections below. But before you dive deeper into inheritance in Python, you’ll take a walk to a dog park to better understand why you might want to use inheritance in your own code.
When creating specific instances of objects, these objects can use the methods defined in the class. In the example below, the fly() method is defined in the IronMan class, and the fly() method is called on the ironMan object. The table shows how classes define the structure for attributes and methods, while objects are individual instances with specific values and behaviours based on the class.
In this example, instead of inheriting from Engine, the Car class is composed with an Engine object, demonstrating the principle of composition over inheritance. In this example, the animal_sound function uses the make_sound method, which behaves differently depending on whether it's called on a Dog or a Cat object. Here, Car is a subclass of Vehicle, inheriting the color and wheels properties and adding a brand property. The State Pattern is used in scenarios where object can exist in multiple states, and the behavior of the object must change dynamically based on its state. This real world example illustrates how the Strategy Pattern allows users to select a specific routing algorithm without needing to understand the inner workings of the algorithm or the application.
Comments
Post a Comment